Every time when you interact with the webpage an interaction will occur we called Event. In this article, we will see the types of events handled in Javascript, and how the event function works.
An event is something that happens when the user interacts with the web page, such as when he clicked a link or button, entered text into an input box, made a selection in a select box, pressed a key on the keyboard, or moves the mouse pointer, or submits the form, etc.
There are other events that the browser triggers, like page load, unload, etc. Events are a part of the Document Object Model (DOM) and every HTML element contains a set of events that can trigger JavaScript Code.
We have different types of events available in Javascript like when a user clicks a button, that click is also an event, same as if we press the keyboard keys, then that keypress too an event.
Let’s discuss more on the Event handlers:
Types of Javascript Events
The events can be categorized into four main groups:
- Mouse Events
- Keyboard Events
- Form Events
- Document/Window Events
Here we will see a few examples to understand the relation between Events and JavaScript.
1. Mouse Events
onclick event:
This event is triggered when the user clicks on any element on a webpage. It can be the link, form button, etc. You can handle click events with an onclick event handler.
For example, The below example will show you an alert after clicking on the button
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | <html> <head> <script> function show() { alert('Hello World!'); } </script> </head> <body> <button type="button" onclick="show()">Click Me</button> </body> </html> |

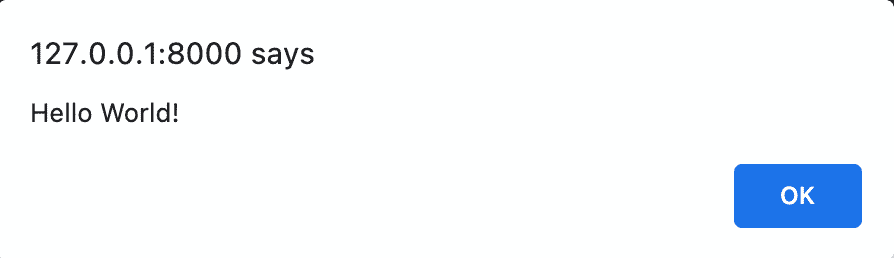
onmouseover event:
The mouseover event occurs when a user moves the mouse pointer over an element. We can handle this event by using onmouseover handler in the element.
1 2 3 | <button type="button" onmouseover="alert('Mouse pointer over a button!');">Place Mouse Over Me</button> |
onmouseout event:
This event is just the reverse of the mouseover event, it occurs when a user moves out the mouse pointer from the element. We can handle this event by using onmouseout handler in the element.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | <html> <head> <script> function show() { alert('Mouse Pointer Outside the button'); } </script> </head> <body> <button type="button" onmouseout="show()">Place Outside</button> </body> </html> |
2. Keyboard Events
onkeydown:
This event is a keyboard event and it executes instructions whenever a key is pressed down.
We can handle the key-down event with the onkeydown event handler.
1 2 3 4 5 | <input type="text" onkeydown="alert('You have pressed a key inside input')"> <textarea onkeydown="alert('You have pressed a key inside textarea')"></textarea> |
onkeyup:
It executes instructions whenever a key is released after pressing.
We can handle the key-up event with the onkeyup event handler.
1 2 3 4 5 | <input type="text" onkeyup="alert('You have released a key inside input')"> <textarea onkeyup="alert('You have released a key inside textarea')"></textarea> |
onkeypress:
This event occurs when the key is pressed down on the keyboard that has a character value associated with it. For example – ctrl, shift, alt, arrow keys, ESC, etc. these keys will not generate the keypress event. But it will trigger onkeyup & onkeydown events.
We can handle the key press event with the onkeypress event handler.
1 2 3 4 5 | <input type="text" onkeypress="alert('You have pressed a key inside input')"> <textarea onkeypress="alert('You have pressed a key inside textarea')"></textarea> |
3. Form Events
A form event is fired when a form control receives or loses control or the user modifies the form control values such as by typing some text, selecting the value from a list, submitting the form, etc.
We will talk about the most used form events below:
onchange:
This event detects when the user changes the value of any form element.
We can handle the change event with the onchange event handler.
1 2 3 4 5 6 7 | <select onchange="alert('You have changed the selection');"> <option>Select</option> <option>Mr.</option> <option>Mrs.</option> </select> |
onfocus:
The focus event occurs when the user gives focus to an element on a web page.
We can handle the focus event with the onfocus event handler. The following example will highlight the border of text input in brown color when it receives the focus.
1 2 3 4 5 6 7 8 9 | <script> function highlight(elm){ elm.style.borderColor = "brown"; } </script> <input type="text" onfocus="highlight(this)"> |

onblur:
This event occurs when the user takes the focus away from the form element or window.
We can handle the event with the onblur event handler. The following example will remove the border of text input when it loses focus.
1 2 3 4 5 6 7 8 9 10 11 12 13 | <script> function blur(elm){ elm.style.borderColor = "none"; } function focus(elm){ elm.style.borderColor = "brown"; } </script> <input type="text" onblur="blur(this)"> <input type="text" onfocus="focus(this)"> |
onsubmit:
This event will be triggered when we submit the form on the webpage. This will take all the input values by default and will submit them to the server or whatever action has been taken.
We can handle the submit event with the onsubmit event handler.
1 2 3 4 5 6 7 | <form action="send.php" method="post" onsubmit="alert('Form data will be submitted.');"> <label>First Name:</label> <input type="text" name="firstname" required> <input type="submit" value="Submit"> </form> |
4. Document/Window Events
Here are some useful events that are triggered on the page loa or when the user resizes the window. Let’s have a look at the most used events below:
onload:
This event is triggered when the page is loaded completely in the web browser.
Let’s understand with the example:
1 2 3 4 5 6 | <body onload="window.alert('Page is loaded successfully');"> <h1>This is a heading</h1> <p>This is paragraph of text.</p> </body> |
onunload:
This event is triggered when a user leaves the current web page by closing the tab or window.
For example:
1 2 3 4 5 6 | <body onunload="window.alert('Are you sure to Exit?');"> <h1>This is a heading</h1> <p>This is paragraph of text.</p> </body> |
onresize:
This event occurs when a user resizes the browser window. The resize event also occurs in situations when the browser window is minimized or maximized.
For example:
1 2 3 4 5 6 7 8 9 10 11 12 13 | <script> function displayWindowSize() { var w = window.outerWidth; var h = window.outerHeight; var txt = "Window size: width=" + w + ", height=" + h; document.getElementById("paragraph").innerHTML = txt; } window.onresize = displayWindowSize; </script> <p id="paragraph"></p> |
Conclusion
So, these all are the events that have specific handlers to trigger the events. One thing that you need to note is that the HTML attribute is not case-sensitive which means you can write in the uppercase, and camelcase also. (like onClick, ONCLICK).
But the function name inside the event is case-sensitive, so you have to make sure after using the events on the elements.
So, these are some of the most frequently used events in JavaScript and their function working. With this, we have come to the end of our article. I hope you understood what are events and how they are used.
For more such content keep following and subscribing to this page. Please reach out to us in the comment section or drop us an email, and we will surely help you in clearing doubts.
Thanks 😉 Happy Learning.
Also, read
- Learn SASS for CSS – A Complete Guide to CSS Preprocessor
- Learn Main Basic Tags in HTML – with examples
- Explain For, While, Do-While Loops in JS and more
- [7] Advanced and Useful Linux – Ubuntu Commands