In this article, we will understand the loops in JS (Javascript), there are different types of loops such as for, while, do…while, etc loops that we are going to learn.
Loops are used in JavaScript to perform repeated tasks based on a condition. The basic idea behind a loop is to automate the repetitive tasks within a program to save time and effort.
For example, if you want to show a message 50 times, then you can use a loop. You can achieve much more with loops.
So, Let’s discuss more on the types of loops and understand with the examples.
Types of Loops in JS (Javascript)
Javascript has five different types of loops:
1️⃣ for Loop: loops through a block of code until the counter reaches a specified number.
2️⃣ while Loop: loops through a block of code as long as the condition specified evaluates to true.
3️⃣ do…while Loop: loops that run a block of code once, then check the condition. If the condition is true, the statement is repeated as long as the specified condition is true.
4️⃣ for…in Loop: loops through the properties of an object.
5️⃣ for…of Loop: loops over iterable objects such as arrays, strings, maps, etc.
✅ For Loop
The for loop repeats a block of code as long as a certain condition is met. It is typically used to execute a block of code a certain number of times. Its syntax is:
1 2 3 4 5 | for (initialization; condition; increment) { // for loop body } |
The for loop consists of three optional expressions, followed by a code block:
- The initialization initializes and/or declares variables and executes only once.
- The condition is evaluated.
- If the condition is false, the for loop is terminated.
- If the condition is true, the block of code inside of the for loop is executed.
- The increment updates the value of initialExpression when the condition is true.
- The condition is evaluated again. This process continues until the condition is false.
If you want to learn more about the conditions, visit here Conditional Statement in JS – with examples.
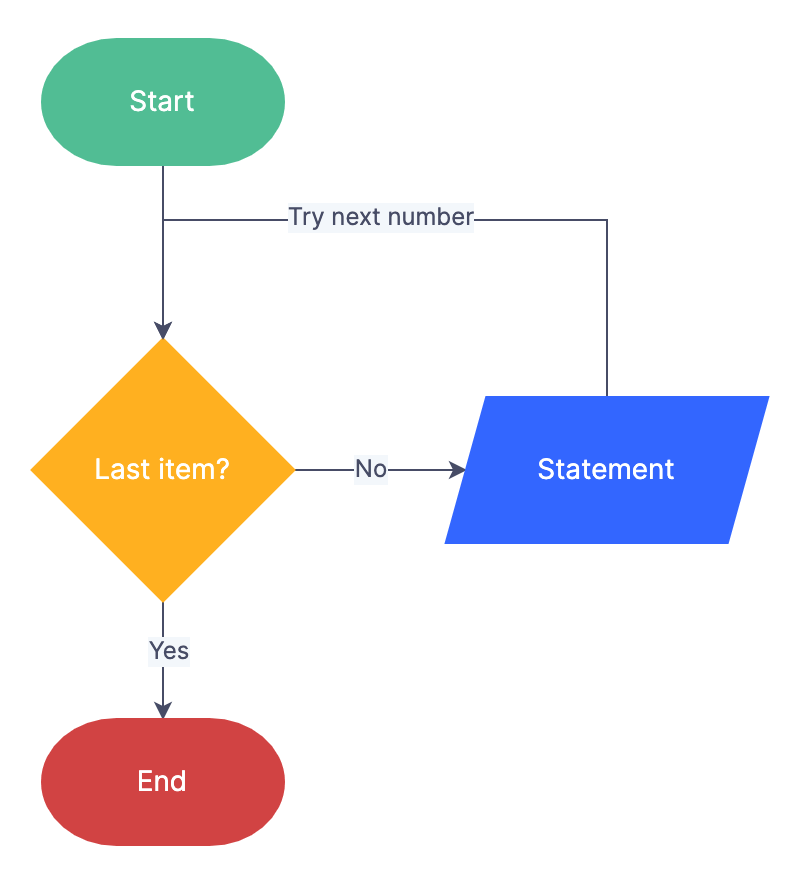
For example: To print the sum of 10 Natural Numbers.
1 2 3 4 5 6 7 8 9 10 11 12 | let n = 4; let sum = 0; // looping from i = 1 to 4 for (let i = 1; i <= n; i++) { sum = sum + i; } console.log('Sum: ' + sum); // Output is Sum: 10 |
Here, the value of the sum is 0 initially. Then, a for loop is iterated from i = 1 to 4. In each iteration, i is added to the sum and its value is increased by 1.
When i become 5, the test condition is false and the sum will be equal to 1 + 2 + 3 + 4.
📔 Note: You can achieve the same result by using the different ways of programming, programming is all about logic.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | // program to display the sum of n natural numbers let sum = 0; let n = 4; // looping from i = n to 1 // in each iteration, i is decreased by 1 for(let i = n; i >= 1; i-- ) { sum = sum + I; } console.log('Sum: ', sum); //Output is Sum: 10 |
✅ While loop
The while loop loops through a block of code as long as the specified condition evaluates to true. As soon as the condition fails, the loop is stopped. The syntax of the while loop is:
1 2 3 4 5 | while(condition) { // while body } |
Here,
- A while loop evaluates the condition inside the parenthesis ( ).
- If the condition evaluates to true, the code inside the while loop is executed.
- The condition is evaluated again.
- This process continues until the condition is false.
- When the condition evaluates to false, the loop stops.
To learn more about the conditions, visit Introduction to Comparison and Logical Operators in JS
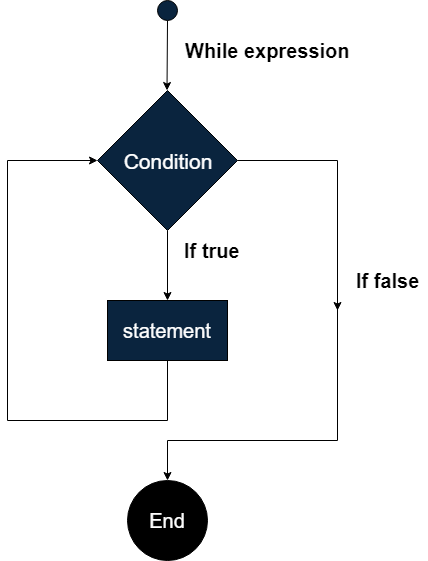
1 2 3 4 5 6 7 8 9 10 11 12 13 | let i = 1; let n = 4; // while loop from i = 1 to 4 while (i <= n) { sum = sum + i; i++; } console.log('Sum: ' + sum); // Output Sum: 10 |
📔 Note: There are several other logic and ways to use the loop to get the same result. The only thing which would be remaining the same is a representation of the while loop.
✅Do…While loop
The do-while loop is a variant of the while loop, which evaluates the condition at the end of each loop iteration.
1 2 3 4 5 6 | do { // body } while(condition); |
See the expression explanation,
- The body of the loop is executed at first. Then the condition is evaluated.
- If the condition evaluates to true, the body of the loop inside the do statement is executed again.
- The condition is evaluated once again.
- If the condition evaluates to true, the body of the loop inside the do statement is executed again.
- This process continues until the condition evaluates to false. Then the loop stops.

Let’s understand with an example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | let i = 1; do { console.log(i); i++; } while(i <= 5); /* Output: 1 2 3 4 5 */ |
It defines a loop that starts with i=1. It will then print the output and increase the value of variable i by 1. After that, the condition is evaluated, and the loop will continue to run as long as the variable i is less than, or equal to 5.
✅ For..in loop
The for...in loop iterates over the properties of an object or the elements of an array. For each property, the code in the code block is executed.
1 2 3 4 5 | for(key in object) { // Code to be executed } |
In each iteration of the loop, a key is assigned to the key variable. The loop continues for all object properties.
- Let’s take an example: Taking object
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | let profile = { name: 'John', class: 6, age: 13 } for (let key in profile) { // display the properties console.log(`${key} => ${profile[key]}`); } // Output // name => John // class => 6 // age => 13 |
📔 Note: The loop counter i.e. key in the for-in loop is a string, not a number. It contains the name of the current property or the index of the current array element.
- You can also use for...in with strings. For example,
1 2 3 4 5 6 7 8 9 10 11 12 13 | let text = 'help'; for (let i in help) { console.log(help[i]); } //Output // h // e // l // p |
- You can also use for...in with arrays. For example,
1 2 3 4 5 6 7 8 9 10 11 12 | let arr = [ 'hello', 2, 'JavaScript' ]; for (let i in arr) { console.log(arr[i]); } //Output // hello // 2 // JavaScript |
📔 Note: You should not use for...in to iterate over an array where the index order is important.
✅ For..of loop
ES6 introduces a new for-of loop that allows us to iterate over arrays or other iterable objects (e.g. strings and special collection types like Set and Map) very easily.
1 2 3 4 5 | for (variable of object) { // code } |
Let’s understand with Examples:
- Iterate over an array
1 2 3 4 5 6 7 8 9 10 11 12 | const arr = [ "One", "Two", "Three" ]; for (let i of arr) { console.log(i); } // Output: // One // Two // Three |
- To Iterate over string
1 2 3 4 5 6 7 | let greet = "Hello World!"; for(let character of greet) { console.log(character); // H,e,l,l,o, ,W,o,r,l,d,! } |
- Using Map
1 2 3 4 5 6 7 8 9 10 11 12 13 | const m = new Map(); m.set(1, "black"); m.set(2, "red"); for (let n of m) { console.log(n); } // Output: // [1, black] // [2, red] |
- Iterate over Set
1 2 3 4 5 6 7 8 9 10 11 12 | const set = new Set([1, 2, 3]); for (let i of set) { console.log(i); } // Output: // 1 // 2 // 3 |
So, this is all about the for, while, do..while, for…in, for…of loops in Javascript (JS). Hope you got to know more about it and I tried to make it easier for you with the examples. Do a lot of practice and use these loops in your projects.
I hope you enjoyed the article and if you found this useful, then please share it with your friends and colleagues. If this post helps you, then spread this so that other people can also benefit.
If you have any queries please feel free to post them in the comments section or anything that you want to ask through mail contact.
Thank you 😉. Happy Coding 🌐
Also read,
- Conditional Statement in JS – with examples
- Introduction to Comparison and Logical Operators in JS
- 5 Useful JavaScript/jQuery codes and techniques with examples – Must use
Great post. I was checking constantly this blog and I’m impressed! Very helpful info particularly the last part 🙂 I care for such information much. I was looking for this certain info for a long time. Thank you and best of luck.