In this post, we will learn how to use the Javascript Slick library to make an interactive and responsive carousel. Slick.js is the Javascript library that uses jQuery to implement the slider/carousel in the component. It is easy to implement.
So, Let’s start and see how anyone can implement it in their projects.
🔹What are the prerequisites of Slick.js ?
Before starting this, we have to import the jQuery library into our project, so that slick can use and help us to make the carousel.
Copy jQuery CDN link for your reference:
1 2 3 | <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js" integrity="sha512-894YE6QWD5I59HgZOGReFYm4dnWc1Qt5NtvYSaNcOP+u1T9qYdvdihz0PPSiiqn/+/3e7Jo4EaG7TubfWGUrMQ==" crossorigin="anonymous" referrerpolicy="no-referrer"></script> |
🔹Include the script and styling
There are two ways to use the script and styling of the Slick slider.
- To download the slick.js and slick.css file from here and copy both files into the project. Then use this below script.
1 2 3 4 5 6 7 | // For script <script src='PROJECT_PATH/js/slick.js'></script> // For Styling <link rel="stylesheet" href="PROJECT_PATH/css/slick.css"> |
- Another way is to use the CDN links that are easy and don’t require any other file.
1 2 3 4 5 6 7 | // For script <script src="https://cdnjs.cloudflare.com/ajax/libs/slick-carousel/1.8.1/slick.min.js" integrity="sha512-XtmMtDEcNz2j7ekrtHvOVR4iwwaD6o/FUJe6+Zq+HgcCsk3kj4uSQQR8weQ2QVj1o0Pk6PwYLohm206ZzNfubg==" crossorigin="anonymous" referrerpolicy="no-referrer"></script> // For Styling <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/slick-carousel/1.8.1/slick.min.css" integrity="sha512-yHknP1/AwR+yx26cB1y0cjvQUMvEa2PFzt1c9LlS4pRQ5NOTZFWbhBig+X9G9eYW/8m0/4OXNx8pxJ6z57x0dw==" crossorigin="anonymous" referrerpolicy="no-referrer"/> |
🔹Let’s understand with the example
Suppose, we have 9 slides to show on the carousel.
1 2 3 4 5 6 7 8 9 10 11 12 13 | <div class="carousel-container"> <div class="slide">Slide-1</div> <div class="slide">Slide-2</div> <div class="slide">Slide-3</div> <div class="slide">Slide-4</div> <div class="slide">Slide-5</div> <div class="slide">Slide-6</div> <div class="slide">Slide-7</div> <div class="slide">Slide-8</div> <div class="slide">Slide-9</div> </div> |
Now, it’s time to initialize the slick to the carousel-container class.
1 2 3 4 5 6 7 8 9 10 | // initialize the slick function $(".carousel-container").slick({ autoplay: true, slidesToShow: 3, slidesToScroll: 1, speed: 350, }); |
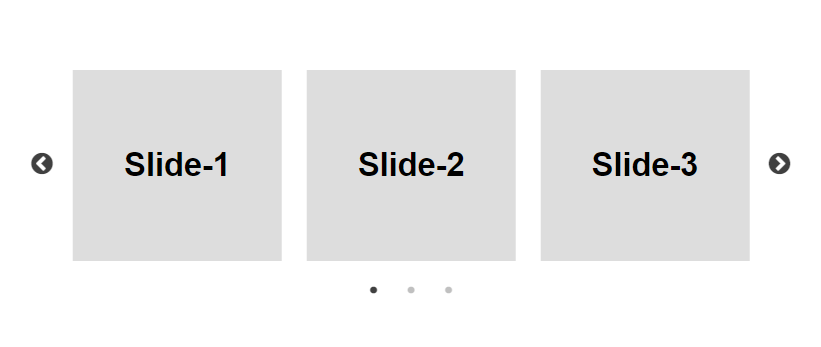
The above piece of code has three slides to show at the same time and one slide scroll with a speed of 350 milliseconds.
We can handle most of the features in the slick, for example, slideToshow, slideToScroll, Dots, etc.
So this is a basic example of how to make a carousel. Now, we will discuss the features to make it a responsive and more cool and interactive carousel.
🔹Cool features of Slick
If we are not passing any parameter in the slick function, then Slick is having predefined properties. For example:
1 2 3 4 5 | // It uses predefined values of slick $('.carousel-container').slick(); |
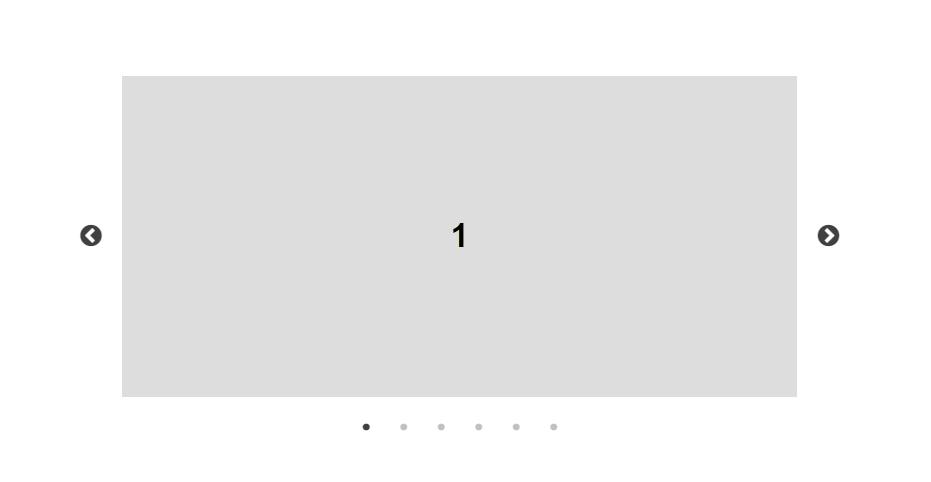
1️⃣ Breakpoints (for responsiveness)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | $('.carousel-container').slick({ dots: true, infinite: true, speed: 350, slidesToShow: 3, slidesToScroll: 3, responsive: [ { breakpoint: 1024, settings: { slidesToShow: 3, slidesToScroll: 3 } }, { breakpoint: 600, settings: { slidesToShow: 2, slidesToScroll: 2 } } ] }); |
So like this, you can customize the features using the responsive, breakpoint properties.
2️⃣ Variable Width
By default, the width of the slides is the same, but if you want to show the real width of the slides, then you can use this property.
1 2 3 4 5 6 7 8 9 | $('.carousel-container').slick({ dots: true, speed: 350, slidesToShow: 3, slidesToScroll: 3, variableWidth: true // use this property }); |

3️⃣ Center Padding
This is one of the cool features of the slick carousel. It helps to highlight the selected slide in the center.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | $('.carousel-container').slick({ centerMode: true, centerPadding: '50px', slidesToShow: 3, responsive: [ { breakpoint: 768, settings: { arrows: false, centerPadding: '40px', } } ] }); |
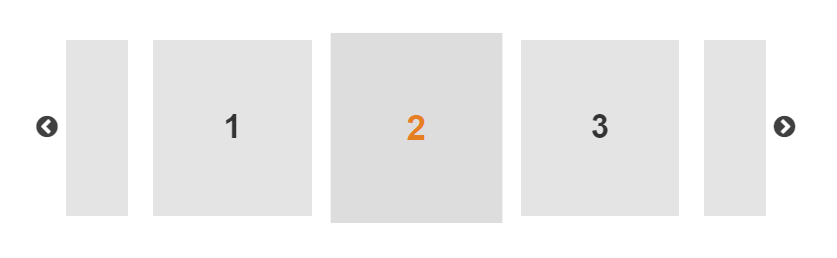
4️⃣ Lazy Loading
Sometimes, we have a requirement in the slider that the image should be loaded for minor seconds and then show the image, this term we could say Lazy loading.
1 2 3 4 5 6 7 8 9 10 | // use data-lazy property instead of src in img <img data-lazy="image/sample1.png"/> $('.carousel-container').slick({ lazyLoad: 'ondemand', slidesToShow: 3, slidesToScroll: 1 }); |
5️⃣ Miscellaneous
- The autoplay property will be used if you want to make the slide in a autoplay mode.
1 2 3 4 5 6 | $('.carousel-container').slick({ autoplay: true, autoplaySpeed: 700, }); |
- If you want to customize the dots, their class then it is also possible.
1 2 3 4 5 6 | $('.carousel-container').slick({ dots: true, dotsClass: 'carousel-pagination', }); |
- Same like dots, if you want to add the selector or class
1 2 3 4 5 6 | $('.carousel-container').slick({ prevArrow: '$('.prev-class')', nextArrow: '$('.next-class')', }); |
- Want to slide in a reverse manner
1 2 3 4 5 | $('.carousel-container').slick({ rtl: true }); |
- To add the fade effect when scrolling
1 2 3 4 5 6 | $('.carousel-container').slick({ fade: true, easing: 'linear', }); |
🔹Some useful Slick functions
You can use these below functions in any of the event listeners of jQuery to customize the slider according to your need.
1 2 3 4 5 | // Destroy the carousel $('.carousel-container').slick('unslick'); |
1 2 3 4 5 | // show the previous slide $('.carousel-container').slick('slickPrev'); |
1 2 3 4 5 | // show the next slide $('.carousel-container').slick('slickNext'); |
1 2 3 4 5 | // it will pause the autoplay $('.carousel-container').slick('slickPause'); |
1 2 3 4 5 | // it will again plays the autoplay $('.carousel-container').slick('slickPlay'); |
So, this is all about the Slick.js library in Javascript / jQuery which you would like to use in your projects. I hope you enjoyed the article and if you found this useful then share this among your friends or on social media.
If you have any queries please feel free to post them in the comments section or anything that you wanted to ask through mail contact.
Thank you😉
Also, read
- 5 Useful JavaScript/jQuery codes and techniques with examples – Must use
- Explain this keyword in JS with examples
- Learn Main Basic Tags in HTML – with examples
- Important Basic Git Commands – Developers should know
- Introduction to Linux – Ubuntu Basic Commands
This is commendable!!
Hi my loved one! I wish to say that this article is
amazing, great written and come with almost all vital infos.
I’d like to peer extra posts like this .
Thanks a lot.
Unquestionably consider that that you stated. Your favorite reason seemed to be at the internet the
simplest thing to consider of. I say to you, I certainly get annoyed whilst other people consider concerns that they plainly
do not recognize about. You managed to hit the nail upon the highest and outlined
out the entire thing with no need side-effects , other
folks can take a signal. Will probably be back to
get more. Thank you
Thanks man!! Happy to see you like it.
This is my first time pay a visit at here and i am in fact happy to read everthing at alone place.
Thanks for reading.